How To Create A Dictionary List In Python
This article was published as a part of the Data Science Blogathon
Python is a high-level general-purpose programming language.Python is one of the most popular and widely used programming languages in the world Python is used for data analytics, machine learning, and even design. Python is used in a wide range of domains owing to its simple yet powerful nature. In this article, we will be discussing lists & dictionaries datatypes.
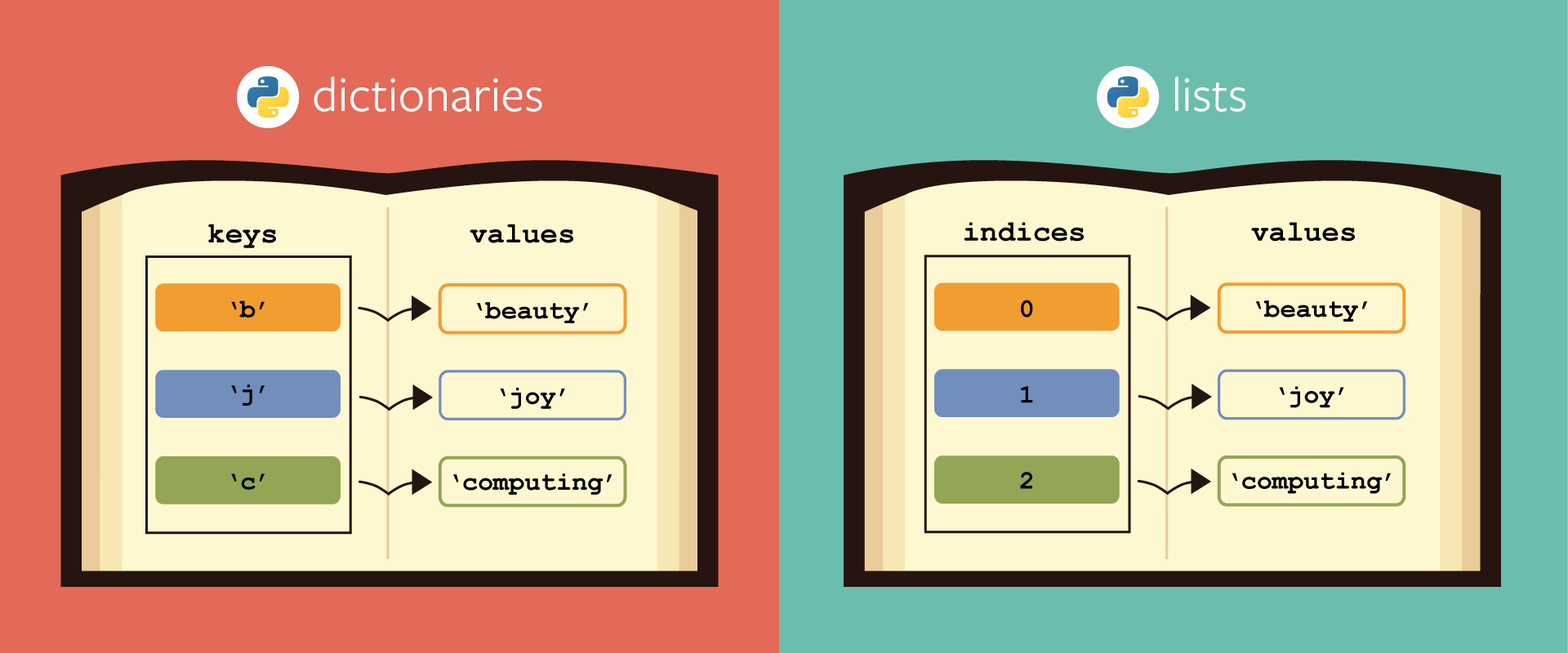
Source: Google Images
Lists
Lists are mutable data types in Python. Lists is a 0 based index datatype meaning the index of the first element starts at 0. Lists are used to store multiple items in a single variable. Lists are one of the 4 data types present in Python i.e. Lists, Dictionary, Tuple & Set.
Accessing the list element
The elements of lists can be done by placing index number in square brackets as shown below
num_list=[10,20,30,40,50,60,70,80,90] city_list=['Agra','Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore']
print(city_list[2])
Output
Ahmedabad
We also have a negative index number as shown below
print(city_list[-1])
Output
Coimbatore
Negative index references the list from the last value, in this case, it is Coimbatore
Index Range
Index range represents the portion of the list that you want to extract as shown in the below example
print(city_list[2:5])
Output
['Ahmedabad', 'Surat', 'Lucknow']
If you want to find the index value of an item in lists provided the item in know, this can be done as shown below
print(city_list.index('Chennai',0,3))
Output
1
Working with operators in List
The addition of two lists can be performed using the + operator. The + operator concatenates both the lists
num_city_list= num_list+ city_list print(num_city_list)
Output
[10, 20, 30, 40, 50, 60, 70, 80, 90, 'Agra', 'Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore']
The * operator is used to extend the list by copying the same values
new_citylist= city_list*2 print(new_citylist)
Output
['Agra', 'Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore', 'Agra', 'Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore']
Nested Lists
A list is nested if another list is present inside it. In the below list there are two elements and both of them are list.
list_nest=[[10,20,30],['a','b','c']] list_nest
Output
[[10, 20, 30], ['a', 'b', 'c']]
The elements of a nested list can be accessed as shown below
print(list_nest[1][2])
Output
c
Updating the lists
In updating the list there is various function. One can append, extend, insert and change the lists. We will see all of them one by one.
Append the lists
Append is used when you want to add one item to the list.
city_list=['Agra','Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore'] city_list.append('Bangalore') print(city_list)
Output
['Agra', 'Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore', 'Bangalore']
Extend the list
Extend is used when you want to add more than one item to the list
num_list=[10,20,30,40,50,60,70,80,90] num_list.extend([100,110,120,130,140,150]) print(num_list)
Output
[10, 20, 30, 40, 50, 60, 70, 80, 90, 100, 110, 120, 130, 140, 150]
Change the lists
Change is used when you want to update a specific item in a list.
num_list[7]= 200 print(num_list)
Output
[10, 20, 30, 40, 50, 60, 70, 200, 90, 100, 110, 120, 130, 140, 150]
Insert in the lists
It is used to insert values at the provided index
city_list=['Agra','Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore'] city_list.insert(4,'Vadodara') print(city_list)
Output
['Agra', 'Chennai', 'Ahmedabad', 'Surat', 'Vadodara', 'Lucknow', 'Coimbatore']
Deleting items in a list
You can remove an item in a list by providing the item index or range that needs to be removed
city_list=['Agra','Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore'] del city_list[4] print(city_list)
Output
['Agra', 'Chennai', 'Ahmedabad', 'Surat', 'Coimbatore']
del city_list[4:6] print(city_list)
Output
['Agra', 'Chennai', 'Ahmedabad', 'Surat']
You can also delete the complete list using the below command
del city_list
Emptying a list
This is very easy to do as shown below
city_list=['Agra','Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore'] city_list=[] print(city_list)
Output
[]
Methods present in a list
Length
Lenght is used to find how many elements are present inside the list
city_list=['Agra','Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore'] print(len(city_list))
Output
6
Clear
Clear is used to delete elements in a list
num_list=[1,2,2,2,3,4,5,6,6,7,6,7] print(num_list.clear())
Output
None
Reverse
The reverse function is used to reverse the elements of a list as shown below
city_list=['Agra','Chennai', 'Ahmedabad', 'Surat', 'Lucknow', 'Coimbatore'] city_list.reverse() print(city_list)
Output
['Coimbatore', 'Lucknow', 'Surat', 'Ahmedabad', 'Chennai', 'Agra']
Sort
Sort is used to sort the elements in a list. By default, it sorts in ascending order, to sort in descending order one parameter needs to be provided in the sort function as shown below
num_list=[1,2,2,2,3,4,5,6,6,7,6,7] num_list.sort(reverse=True) print(num_list)
Output
[7, 7, 6, 6, 6, 5, 4, 3, 2, 2, 2, 1]
Dictionary
Dictionaries are mutable data types in nature meaning they can be updated after they are created. Syntactically they are written in a key, value pair format inside curly braces.
{Key1:Value1,Key2:Value2,Key3:Value3,Key4:Value4}
Keys are always unique and there cannot be any duplicates. There is no index in the dictionary meaning they are not ordered. Key is the default iterator and is used to retrieve the value.
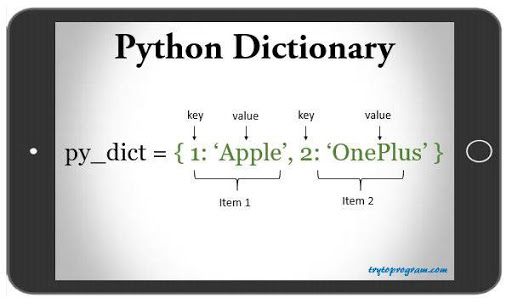
Source: Try to Program
How a Dictionary is created?
There are four ways in which a dictionary can be created
1) Empty Dictionary
dict_emp={} print(dict_emp)
Output:
{}
2) When keys are integer values
dict_city={1: 'Ahmedabad', 2: 'Chennai', 3: 'Coimbatore',4:'Surat',5:'Agra'} print(dict_city)
Output
{1: 'Ahmedabad', 2: 'Chennai', 3: 'Coimbatore', 4: 'Surat', 5: 'Agra'}
3) When keys are string values
dict_travel={'Country1':'USA', 'Country2': 'India', 'Country3':'Japan', 'Country4':'UK', 'Country5': 'Australia'} print(dict_travel)
Output
{'Country1': 'USA', 'Country2': 'India', 'Country3': 'Japan', 'Country4': 'UK', 'Country5': 'Australia'}
4) When mixed keys are present
mixed_dict= {'Country':'India', 1456:'Free','list':['city', 'road',12]} print(mixed_dict)
Output
{'Country': 'India', 1456: 'Free', 'list': ['city', 'road', 12]}
Different ways to access list elements
Let's create two dictionary
dict_salesid= {'SID1': Fiat 'SID2': Mercedes 'SID3': Maruti 'SID4': Volkswagen 'SID5': Kia}
dict_salesinfo= {'SID':Fiat, 'Sales': 20000 'LaunchDay':'Wed' 'Cost': 500000}
Length of the dictionary
print(len(dict_salesid))
Output
5
Extracting dictionary element using its key
sales_id='SID2' if sales_id in dict_salesid: name= dict_salesid[sales_id] print('Sales ID is {}, Sales name is {}'. format(sales_id,name)) else: print('Sales ID {} not found'.format(sales_id))
Output
Sales ID is SID2, Sales name is Mercedes
Setting dictionary element using its key
First we have set the dictionary element and then we have retrieved it using get function
dict_salesinfo['LaunchDay']='Thurs' dict_salesinfo['Cost']=6000000 LaunchDay= dict_salesinfo.get('LaunchDay') Cost=dict_salesinfo.get('Cost') print('Launchday is {}, Cost is {}'. format(LaunchDay,Cost))
Output
Launchday is Thurs, Cost is 6000000
Delete
A dictionary object can be deleted using the del statement
del dict_salesinfo
Output:
The dictionary object is deleted
Deleting a specific item
To delete a specific item pass on the dictionary key in the del statement as shown below
del dict_salesinfo['SID'] print(dict_salesinfo)
Output
{'Sales': 20000, 'LaunchDay': 'Wed', 'Cost': 500000}
Another way to delete a specific item is using the pop function
print(dict_salesinfo.pop('SID'))
Output
Fiat
The pop function also returns the key value that is being deleted. In this case, it is Fiat.
The third method to delete a dictionary object is using the clear method
print(dict_salesinfo.clear())
Output
None
Looping through a dictionary object
We will use the same dictionary object dict_salesinfo. Using the keys function first save all the keys in a dict_key variable
dict_keys= dict_salesinfo.keys()
print(dict_keys)
print(type(dict_keys))
Output
dict_keys(['SID', 'Sales', 'LaunchDay', 'Cost']) <class 'dict_keys'>
for var in dict_keys:
print(var + ":" + str(dict_salesinfo[var]))
Output
SID:Fiat Sales:20000 LaunchDay:Wed Cost:500000
Printing dictionary object values in key, value pair
dict_values= dict_salesinfo.values() print(dict_values)
Output
dict_values(['Fiat', 20000, 'Wed', 500000])
Using the items() function
The item function converts a dictionary item into a tuple
dict_items= dict_salesinfo.items() print(dict_items) print(type(dict_items))
Output
dict_items([('SID', 'Fiat'), ('Sales', 20000), ('LaunchDay', 'Wed'), ('Cost', 500000)]) <class 'dict_items'>
Looping through the items function
for key, value in dict_salesinfo.items(): print(key +"-"+str(value))
Output
SID-Fiat Sales-20000 LaunchDay-Wed Cost-500000
Converting a list into a dictionary object
Dictionary object contains key-value pairs, and the list must adhere to this format or else it will throw an error.
sales_infolist=[['SID','Fiat'],['Sales','20000'],['LaunchDay','Wed'],['Cost','500000']] print(type(sales_infolist)) sales_infolist_dict= dict(sales_infolist) print(type(sales_infolist_dict))
Output
<class 'list'> <class 'dict'>
Copying a dictionary into a new dictionary
dict_salesinfo_new= dict_salesinfo.copy() print(dict_salesinfo_new)
Output
{'SID': 'Fiat', 'Sales': 20000, 'LaunchDay': 'Wed', 'Cost': 500000}
Updating the dictionary object
The update method is used to update the dictionary object with a
dict_salesinfo= {'SID':'Fiat','Sales': 20000,'LaunchDay':'Wed','Cost': 500000} dict_salesinfo.update({'Profit':'50000'}) print(dict_salesinfo)
Output
{'SID': 'Fiat', 'Sales': 20000, 'LaunchDay': 'Wed', 'Cost': 500000, 'Profit': '50000'}
I hope you enjoyed reading, and feel free to use my code to try it out for your purposes. Also, if there is any feedback on code or just the blog post, feel free to email me at [email protected]
The media shown in this article are not owned by Analytics Vidhya and are used at the Author's discretion.
How To Create A Dictionary List In Python
Source: https://www.analyticsvidhya.com/blog/2021/06/working-with-lists-dictionaries-in-python/
Posted by: solistheaks.blogspot.com
0 Response to "How To Create A Dictionary List In Python"
Post a Comment